iText 7 ASP.NET Core: Solve “FontProvider and FontSet are Empty” Error
In the realm of web development, PDF generation is a crucial aspect, often requiring the integration of third-party libraries. When working with ASP.NET 6 and iText 7 for PDF creation, developers may encounter the perplexing “FontProvider and FontSet are Empty” error. This blog post aims to shed light on this issue and provide a step-by-step guide on how to resolve it.
Understanding the Error
The “FontProvider and FontSet are Empty” error can be a stumbling block for developers attempting to generate PDF content using iText 7 in an ASP.NET 6 application. This error typically indicates a lack of font information for the PDF document, causing the library to struggle with font resolution during the rendering process.
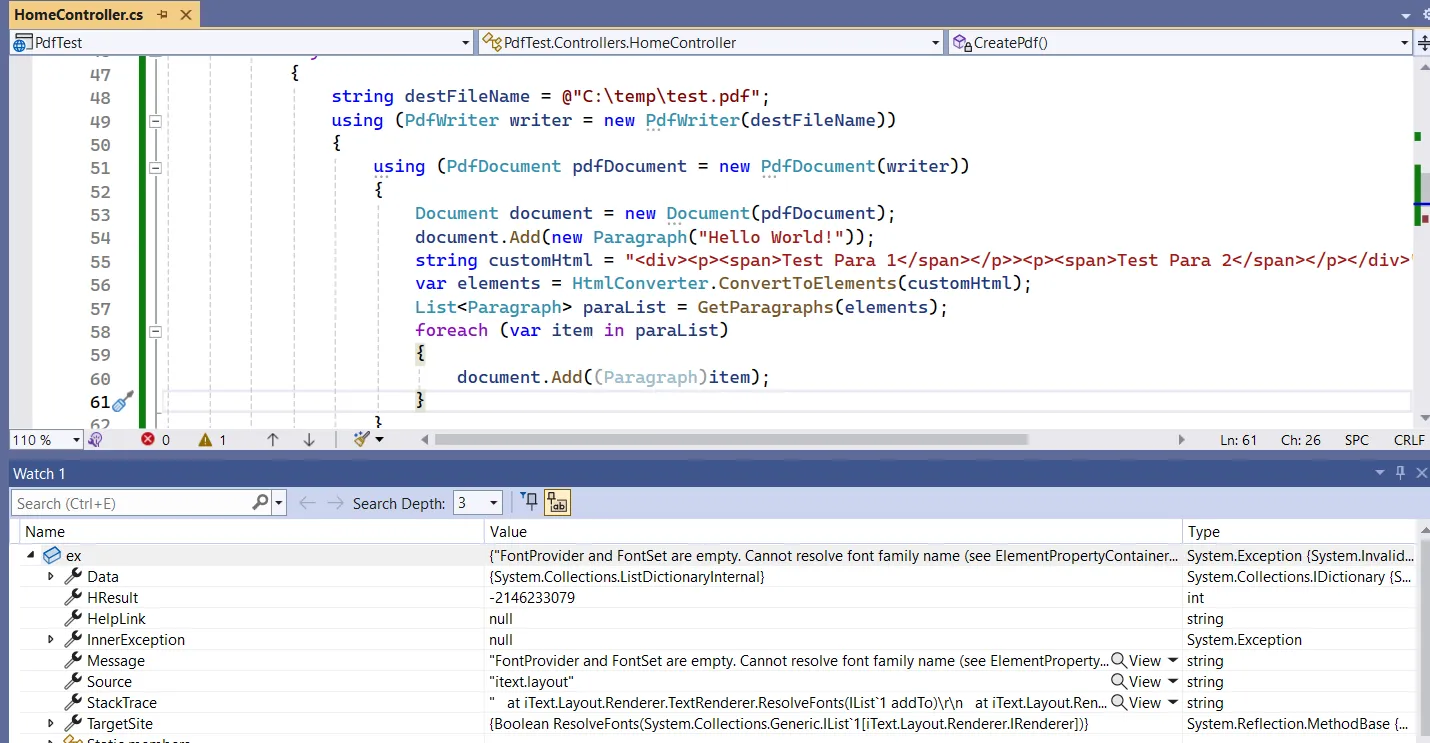
Application will break at “document.add(item)”
Identifying the Root Cause
To address this error, it’s crucial to delve into its origins. Often, it stems from font properties not being properly set within the HTML content that you are attempting to convert into a PDF. Fonts play a pivotal role in the appearance of the document, and iText 7 requires accurate font information for seamless PDF generation.
When we checked the “Font” property of every “item”, we found that it is set to the “times” string and there is no font available in the “StandardFonts” constants as well.
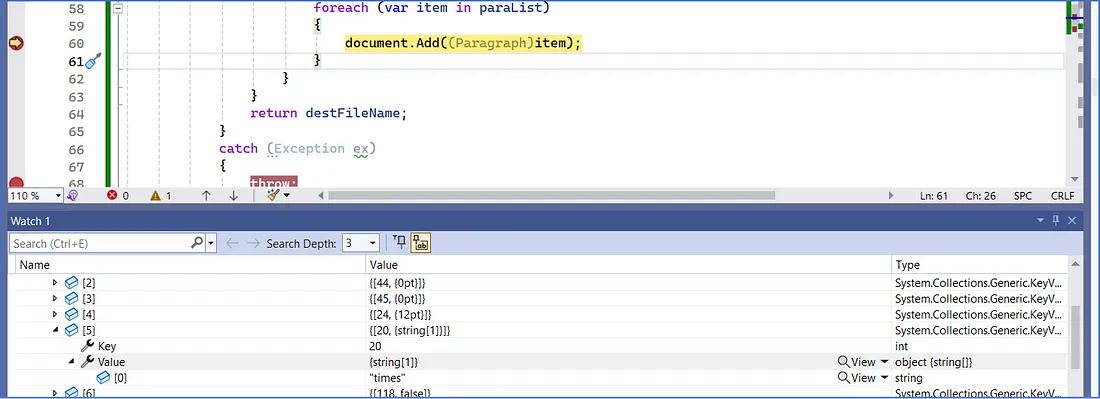
Notice that “item” has “times” as font property.
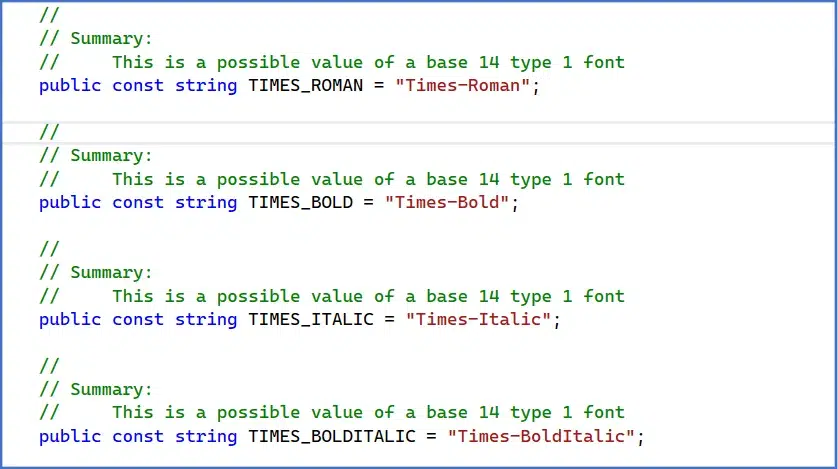
In the “StandardFonts” class “times” is not defined.
Solving the Issue — A DIY Approach
One effective solution to the “FontProvider and FontSet are Empty” error involves creating a function to remove font properties from all nested elements in the HTML content. By systematically cleaning up the font-related attributes, developers can ensure a clean slate for the PDF generation process. It will follow the parent element/document font style. This DIY approach tackles the issue head-on, providing a workaround while maintaining the structural integrity of the HTML content.
if (element.HasProperty(Property.FONT))
{
element.DeleteOwnProperty(Property.FONT);
}
Step-by-Step Guide
In this section, we will provide a step-by-step guide on implementing the function to remove font properties. This guide will empower developers to address the error efficiently, allowing for a smooth PDF generation process in their ASP.NET 6 applications using iText 7.
Step 1: Create a Windows/Web project in .Net 6 (We have created an Asp.Net MVC project)
Step 2: Add “itext7”, “itext7.pdfhtml”, “itext.bouncy-castle-adapter” Nuget libraries
Step 3: Add the below code in the “HomeController” class and add the “var data = CreatePdf();” code in the “Index()” method.
private string CreatePdf()
{
try
{
string destFileName = @"C:\temp\test.pdf";
using (PdfWriter writer = new PdfWriter(destFileName))
{
using (PdfDocument pdfDocument = new PdfDocument(writer))
{
Document document = new Document(pdfDocument);
document.Add(new Paragraph("Hello World!"));
string customHtml = "<div><p><span>Test Para 1</span></p> > <p><span>Test Para 2</span></p></div>";
var elements = HtmlConverter.ConvertToElements(customHtml);
List<Paragraph> paraList = GetParagraphs(elements);
foreach (var item in paraList)
{
document.Add((Paragraph)item);
}
}
}
return destFileName;
}
catch (Exception ex)
{
throw;
}
}
private List GetParagraphs(IList elements)
{
List<Paragraph> list = new List<Paragraph>();
foreach (IElement element in elements)
{
if (element is Paragraph)
{
list.Add((Paragraph)element);
}
else if (element is IBlockElement)
{
var tempList = GetParagraphs(((IBlockElement)element).GetChildren());
if (tempList.Count > 0)
{
list.AddRange(tempList);
}
}
}
return list;
}
Step 4: Now run the project. You will face the “FontProvider and FontSet are Empty” error.
Step 5: Stop the debugging and add the below code. Also add “ClearUnwantedProperties(elements);” this code after “HtmlConverter.ConvertToElements(customHtml);” in “CreatePdf()” function.
private void ClearUnwantedProperties(IList elements)
{
foreach (IElement element in elements)
{
if (element.HasProperty(Property.FONT))
{
element.DeleteOwnProperty(Property.FONT);
}
if (element is IBlockElement)
{
var childs = ((IBlockElement)element).GetChildren();
if (childs.Count > 0)
{
ClearUnwantedProperties(childs);
}
}
}
}
Now run the code. The issue is resolved! Happy Coding!
Alternative Solution:
Another approach is you add FontProvider for font “times” so you will not need to remove Font property. But we have not followed this approach because today “HtmlConverter.ConvertToElements” applies the “times” string and it may be different for other elements or in the future. So instead we removed the font property and followed our standard fonts.
Best Practices for PDF Generation in ASP.NET 6
To prevent similar font-related issues in the future, adopting best practices for PDF generation is essential. This section will explore recommendations for handling fonts, styling, and other nuances that contribute to a seamless and error-free PDF generation experience.
Conclusion:
Resolving the “FontProvider and FontSet are Empty” error in iText 7 for PDF generation in ASP.NET 6 requires a strategic approach. By understanding the error, identifying its root cause, and implementing a practical solution, developers can overcome this obstacle and enhance the reliability of their PDF generation process. This blog post aims to equip developers with the knowledge and tools needed to tackle font-related challenges and deliver polished PDF documents in their ASP.NET 6 applications.