Most Commonly Found Vulnerabilities in Web Applications.
React.js, Next.js, and Express.js are widely used frameworks for developing dynamic, modern web applications. While these technologies offer powerful tools for developers, they do not inherently guarantee the security of an application. It is essential to understand and address common vulnerabilities within this technology stack to ensure the development of secure and resilient software.
Cross-Site Scripting (XSS) Attacks:
Reflected XSS (via GET Parameters):
Reflected XSS attacks occur when malicious scripts are injected through URL parameters (GET requests). These scripts can steal sensitive data, such as cookies, or redirect users to attacker-controlled websites with minimal user interaction. This vulnerability is typically considered a medium to high-level threat and is exploited through improperly sanitized GET parameters.
Stored XSS (via POST Parameters):
Stored XSS attacks are more severe, injecting malicious code through POST parameters, which are then stored in a database. When this data is retrieved and displayed on the front end, the malicious payload is executed. This can lead to the theft of cookies, session hijacking, or redirection to malicious websites, often with no user interaction required. Stored XSS is considered a high to critical-level vulnerability and requires strong input validation and output encoding mechanisms to mitigate.
Example payload to test for Cross-Site Scripting Attacks:
· To create a popup alert: “><img src=x onerror=alert(3);//>
· To redirect a user to other domains: “><img src=x onerror=location.replace(“https://attacker-controlled-domain”);//>
Use Case Scenario: Cross-Site Scripting (XSS) Vulnerabilities in GET and POST Parameters
In both GET and POST request methods, web applications may be vulnerable to Cross-Site Scripting (XSS) attacks if user-supplied data is not properly validated and sanitized. This can occur when an attacker injects malicious script code into a parameter value that is later reflected or stored by the server and executed on the client side.
Proof Of Concept:
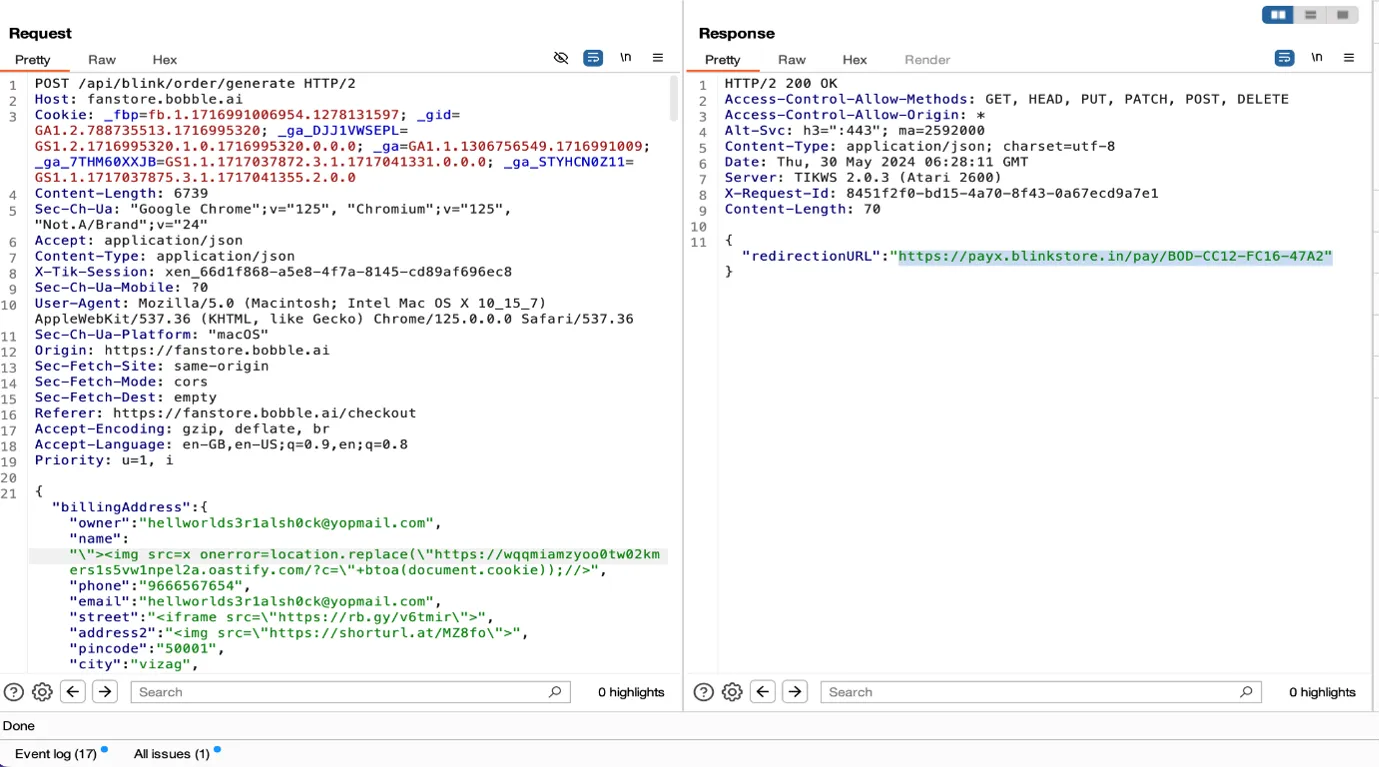
Cross Site Payload that is being executed based of the malicious user input.
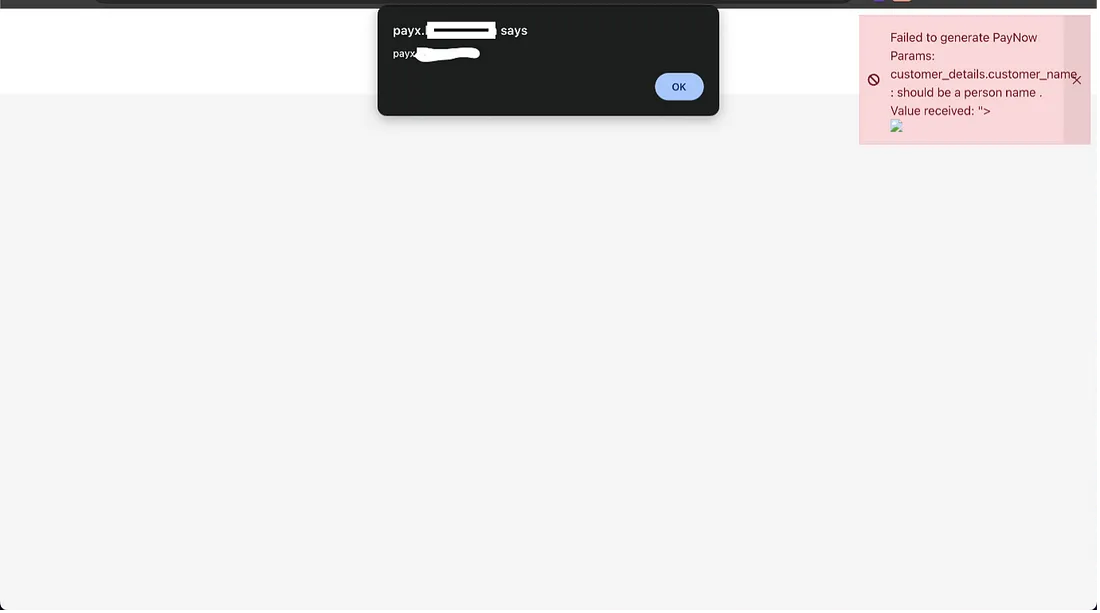
Solution to Prevent Cross-Site Scripting (XSS) Attacks
The most effective way to mitigate Cross-Site Scripting (XSS) attacks is to HTML encode user input before storing it in the database. This process ensures that any potentially harmful scripts are treated as plain text, rather than executable code. By encoding user input, the application safeguards against the execution of malicious payloads when data is retrieved and rendered on the frontend.
- Input Sanitization and Encoding: When accepting user input, it is crucial to sanitize and encode special characters (e.g., <, >, &, and “), transforming them into their respective HTML entities. This prevents the input from being interpreted as HTML or JavaScript by the browser.
- Storage and Retrieval: By storing the encoded data in the database, you ensure that any potentially dangerous input is stored as harmless text. When the data is retrieved and rendered on the frontend, the encoded characters are displayed as intended — without triggering any scripts or payloads.
This approach nullifies the risk of stored XSS attacks, as the payloads stored in the database are never executed when the data is displayed to the user.
Indirect Object Reference (IDOR) Vulnerabilities:
Indirect Object Reference (IDOR) vulnerabilities occur when an attacker can access or modify data that they should not be authorized to view, typically by manipulating parameters, such as auserId, in the URL or request. These vulnerabilities arise when an application does not properly validate whether the requesting user has permission to access the specified resource.
- Attack Mechanism: An attacker can often exploit IDOR by modifying the userId parameter (or a similar identifier) to reference the account or data of another user. In many cases, attackers may attempt to brute-force the userId values, particularly when there is no specific target in mind and the attacker is simply trying to access data indiscriminately. This can lead to unauthorized access to sensitive user information.
- Example: An attacker could change the userId=123 parameter in a request URL to userId=124, allowing them to retrieve another user’s data, if the application does not adequately enforce authorization checks.
Authentication and Authorization Issues in APIs:
Lack of Authentication on API Endpoints:
In many cases, API endpoints are not properly secured, allowing attackers to access sensitive information without proper authentication. This leaves the API exposed, with the potential for unauthorized users to exploit it. If sensitive data, such as personal information or credentials, is exposed through these unprotected endpoints, attackers can easily steal or manipulate this information.
Authorization Bypass (Privilege Escalation):
- Even if API endpoints require authentication, they may not properly check if the authenticated user has the appropriate authorization to access specific resources. For instance, a normal user might be able to access admin-level endpoints if the application only checks for authentication and not for the user’s roles or privileges.
- Example: A user who is authenticated could, inappropriately, access an endpoint designed for administrators by simply knowing the endpoint URL, even though they do not have the necessary privileges to perform such actions.
In this example, we can see that just by changing the userId we are able to fetch data of other users.
Proof Of Concept:
Solution to Prevent IDOR’s
To address this issue, developers should ensure that all API endpoints requiring access to sensitive data are protected by authentication mechanisms, such as JWT (JSON Web Tokens). These tokens should encapsulate both the user’s authentication and authorization details, enabling the server to validate not only the identity of the user but also their permissions to access specific endpoints. By enforcing token-based authentication and verifying the user’s roles and privileges, developers can effectively secure API endpoints and prevent unauthorized access.
Logical Bugs:
Business logic vulnerabilities are often subtle and challenging to identify, as they typically arise from flaws in the way an application processes input to generate output. In many cases, developers implement logic to automate certain processes without thoroughly validating or sanitizing user input before it is used. This oversight creates opportunities for attackers to exploit the system by providing unexpected input that bypasses intended controls.
Example of Exploit: An attacker may manipulate a quantity field by entering a value like “0.1”, which could lead to a pricing error or unintended discount, resulting in financial gain. This is a classic example of faulty business logic, where improper validation allows users to manipulate critical application processes.
Proof Of Concept:
A solution to the Problem:
To address business logic vulnerabilities, developers should implement strict input validation rules to ensure that user-provided data matches the expected data types and formats. By validating input before processing, the application can enforce proper constraints and prevent unintended manipulation of business processes. This simple yet effective check will help eliminate logic flaws and ensure the application generates correct, expected results.
HTML Injection Attacks:
HTML injection attacks occur when an application fails to properly sanitize user input, allowing attackers to inject malicious HTML tags — such as <a> (anchor) or <iframe> tags—into the application. These injected tags can redirect users to attacker-controlled websites, expose sensitive information such as IP addresses, or perform other harmful actions. The injected HTML is often stored in the database through POST parameters, and when the affected content is later rendered, the malicious payloads are executed in the browsers of unsuspecting users. This creates significant security risks, as it enables attackers to exploit the application’s trust in user-generated content.
Payload: “><iframe src=”https://attacker-controlled-domain.com”;//>
Use case scenario: Use the payload in any post parameter where the value of the post parameter will be saved in the database.
Proof Of Concept:
A solution to the Problem:
To prevent HTML injection attacks, it is crucial to HTML encode user input before storing it in the database. This ensures that any potentially harmful HTML tags or scripts are treated as plain text rather than executable code. By following this workflow, the application can safely store and display user input, preventing malicious payloads from being triggered on the front end when the data is rendered.
Vulnerable and Outdated JavaScript Libraries and Frameworks:
Vulnerabilities often arise when developers use outdated JavaScript libraries and frameworks, focusing solely on achieving the desired functionality without considering potential security risks. These outdated libraries may contain known vulnerabilities or CVEs (Common Vulnerabilities and Exposures), which can be exploited by attackers to carry out malicious activities. Failure to regularly update and patch these dependencies exposes applications to security threats that can compromise their integrity and safety.
Proof Of Concept:
A solution to the Problem:
To mitigate the risks associated with outdated JavaScript libraries and frameworks, always use the latest stable release of the framework or library in use. If an older version is necessary due to compatibility or other constraints, ensure that any security patches or updates addressing known CVEs (Common Vulnerabilities and Exposures) are promptly implemented. Regularly monitor for new security advisories and update dependencies as needed to reduce the potential for exploitation.